Android is an open source operating system that’s based on Linux. Android applications are packaged as APK (Android Package Kit) files. These files contain all the elements an application needs to function correctly.
This article will be looking at common Android application vulnerabilities, and tools that can be used to identify and exploit them.
Android Security Basics
Android implements a number of security mechanisms.
Application Signing: Applications must be cryptographically signed before being installed on an Android device.
Application Sandboxing: Each Android application runs in it’s own environment so it’s isolated from other applications and the system.
Permissions: Applications must explicitly request permissions to access certain resources or data, such as the camera, location and contacts. This is can done at installation time or runtime.
Content Providers: Content providers manage access to shared data. Apps must declare which content providers they expose, and other apps need permission to access those providers.
Intents: Intents allow communication between different applications and components. To avoid malicious interception or hijacking, intent filters are used to specify which apps can respond to certain intents.
Certificate Pinning: Certificate pinning is a security mechanism that ensures only certificates that are embedded into an application are accepted when making a secure connection.
Common Android Security Issues
OWASP track the top 10 mobile security risks in the OWASP Mobile Top 10:2024. A summary of these issues can be found below.
# | Risk | Description | Example | Mitigation |
---|---|---|---|---|
1 | Improper Credential Usage | Flaws related to the mishandling of credentials within mobile applications. | Hard-coded credentials, improper storage of user credentials. | Avoid hard coding credentials, securely store credentials using platform-specific secure storage mechanisms. |
2 | Inadequate Supply Chain Security | Vulnerabilities arising from insecure components and dependencies within the mobile app’s supply chain. | Use of outdated libraries, insecure third-party services. | Regularly update and patch components, conduct thorough security assessments of third-party services. |
3 | Insecure Authentication/Authorization | Weaknesses in authentication and authorisation mechanisms leading to unauthorised access. | Insufficient password policies, improper session management. | Implement strong authentication methods, enforce robust password policies, and manage sessions securely. |
4 | Insufficient Input/Output Validation | Lack of proper validation of input and output data, leading to potential vulnerabilities. | Accepting invalidated user inputs, improper data sanitisation. | Validate and sanitise all user inputs and outputs to prevent injection attacks and data corruption. |
5 | Insecure Communication | Flaws in securing data transmitted over networks, leading to potential interception and tampering. | Sending sensitive data over unencrypted channels. | Use strong encryption protocols (e.g., TLS) for all data transmissions. |
6 | Inadequate Privacy Controls | Insufficient mechanisms to protect user privacy and sensitive data. | Collecting excessive user data without consent, improper handling of personal information. | Implement data minimisation principles, obtain user consent, and ensure compliance with privacy regulations. |
7 | Insufficient Binary Protections | Weaknesses in protecting the app’s binary code from reverse engineering and tampering. | Lack of code obfuscation, absence of integrity checks. | Use code obfuscation techniques, implement integrity checks, and secure the app’s binary code. |
8 | Security Mis-configuration | Insecure default configurations and incomplete setups leading to vulnerabilities. | Leaving default credentials unchanged, unnecessary services running. | Regularly review and update configurations, disable unused services, and handle errors securely. |
9 | Insecure Data Storage | Storing sensitive data insecurely on the device. | Storing passwords, API keys, or sensitive data in plain text. | Use strong encryption to store sensitive data. Utilise platform-specific secure storage mechanisms. |
10 | Insufficient Cryptography | Weak or improper cryptographic algorithms and key management exposing sensitive data. | Using outdated encryption algorithms or storing encryption keys insecurely. | Use strong, industry-standard cryptographic algorithms and securely manage encryption keys. |
Development Tools
To investigate the security of an Android application, we’re going to need some tools.
We will be using Ubuntu as our host operating system. Although you can install these tools inside a Virtual Machine, performance running an Android emulator will be poor.
Android Studio – This is the official Integrated Development Environment (IDE) provided by Google. This can easily be installed through the Ubuntu software centre.
In addition, the following apt packages will be required.
APT Package | Purpose |
---|---|
android-sdk-platform-tools-common | This package typically contains the core set of platform tools required for interacting with Android devices, such as the ADB (Android Debug Bridge) and fastboot tools. |
aapt | Android asset packaging tool |
apktool | Tool for reverse engineering APK files |
default-jdk | Java Development Kit |
These can be installed with;
sudo apt install android-sdk-platform-tools-common aapt apktool default-jdk
To ensure these tools are working correctly, we will be using a deliberatly vulnerable application, InjuredAndroid that can be downloaded from GitHub.
Uploading & Downloading APK Files
Once you have an emulator configured in Android Studio, your going to want the ability to upload and download APK files to it.
The Android Asset Packaging Tool (AAPT) can be used to determine the CPU architecture an APK file targets. The APK file can then be installed using the Android Debug Bridge (ADB).
user@host:~$ aapt dump badging InjuredAndroid-1.0.12-release.apk | grep native-code
native-code: 'arm64-v8a' 'armeabi-v7a' 'x86_64'
user@host:~$ adb install InjuredAndroid-1.0.12-release.apk
Performing Streamed Install
Success
To extract an APK file from an existing device, we can use the adb pull command.
user@host:~$ adb shell
emu64xa16k:/ $
emu64xa16k:/ $ pm list packages | grep inj
package:b3nac.injuredandroid
emu64xa16k:/ $ pm path b3nac.injuredandroid
package:/data/app/~~x_blJzdZ_3626bg44TBMMw==/b3nac.injuredandroid-r-J9eUcXQDy-FJahCwEnLA==/base.apk
user@host:~$ adb pull /data/app/~~x_blJzdZ_3626bg44TBMMw==/b3nac.injuredandroid-r-J9eUcXQDy-FJahCwEnLA==/base.apk apk_backup.apk
/data/app/~~x_blJzdZ_3626bg44TBMMw==/b3nac.injuredandroid-r-J9eUcXQDy-FJahCwE...A==/base.apk: 1 file pulled, 0 skipped. 154.6 MB/s (24725001 bytes in 0.153s)
JADX-GUI
Most Android applications are written in Java, or Kotlin. Kotlin is a newer language that recommended for Android development by Google. Both of these languages can be decompiled using JADX-GUI.
JADX-GUI is a tool to decompile Dalvik Executable (DEX) to source code. DEX files are compiled Java byte code optimised for execution on the Android platform by the Dalvik or Android Runtime (ART) virtual machines.
Download the latest release from here: https://github.com/skylot/jadx/releases.
Opening a APK file with JADX-GUI, we can navigate the applications source code.
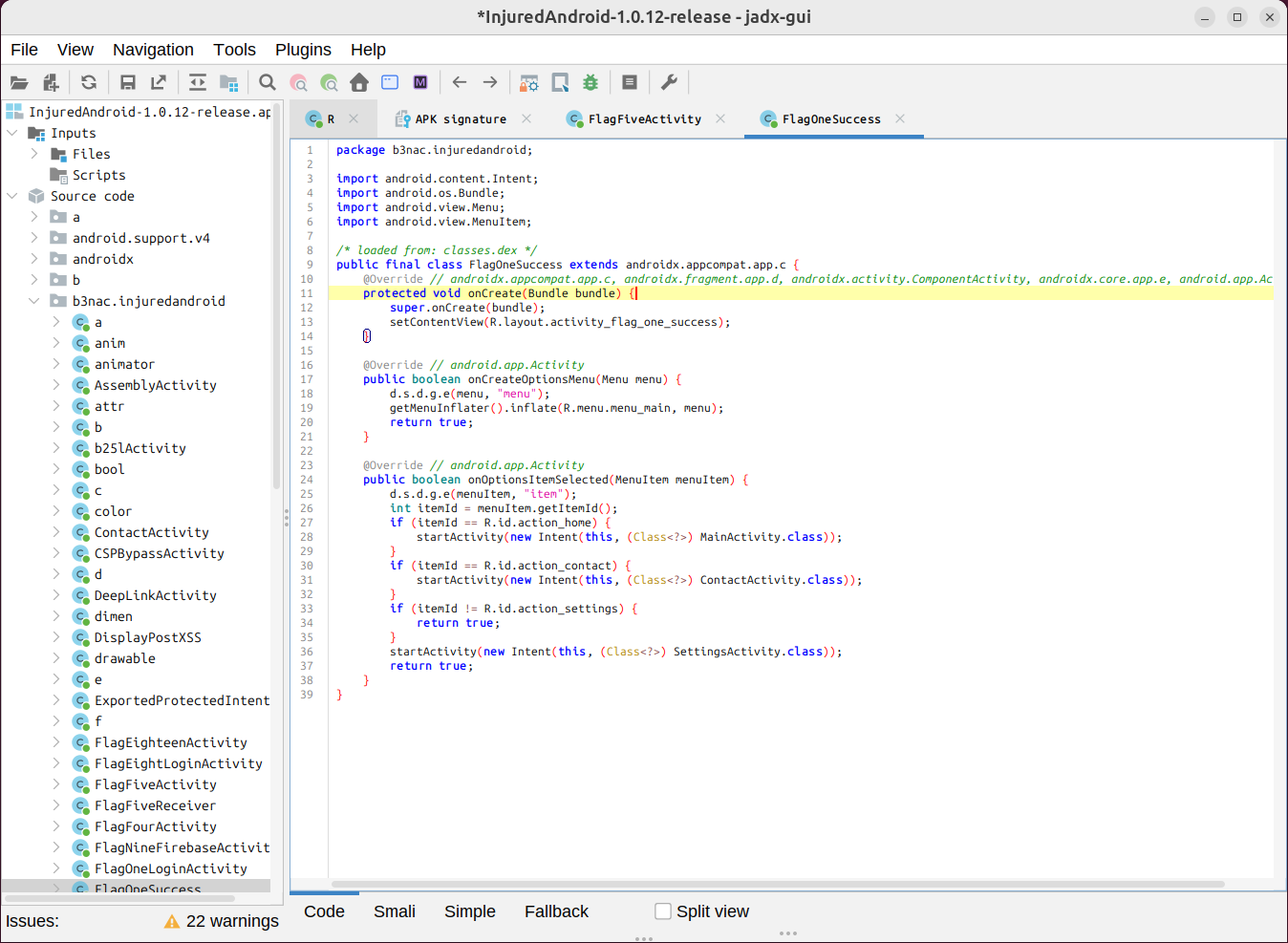
The code won’t be an exact one to one replication of the original source code, but should be close enough to assist in identifying vulnerabilities.
APK File Extraction
In addition to using JADX-GUI we can use APKTool to unpack the contents of an APK file and manually examine it’s contents.
user@host:~/Android/APK$ apktool d InjuredAndroid-1.0.12-release.apk
I: Using Apktool 2.7.0-dirty on InjuredAndroid-1.0.12-release.apk
I: Loading resource table...
I: Decoding AndroidManifest.xml with resources...
I: Loading resource table from file: /home/user/.local/share/apktool/framework/1.apk
I: Regular manifest package...
I: Decoding file-resources...
I: Decoding values */* XMLs...
I: Baksmaling classes.dex...
I: Copying assets and libs...
I: Copying unknown files...
I: Copying original files...
I: Copying META-INF/services directory
Embedded Secrets
Strings in an Android application are typically stored in a file called strings strings.xml. With this file extracted from an APK, we can search the contents for stored credentials.
~/Android/APK/InjuredAndroid-1.0.12-release$ cat ./res/values/strings.xml | grep -i api_key
<string name="google_api_key">AIzaSyCUImEIOSvqAswLqFak75xhskkB6illd7A</string>
<string name="google_crash_reporting_api_key">AIzaSyCUImEIOSvqAswLqFak75xhskkB6illd7A</string>
Alternatively, the tool APKLeaks can be used to identify stored API keys and credentials. Install using;
git clone https://github.com/dwisiswant0/apkleaks
sudo apt install python3-pyaxmlparser
Running this against InjuredAndroid shows an embedded Google API Key. This API key is used to authenticate an application against Google’s online services.
python3 apkleaks.py -f /home/user/Android/APK/InjuredAndroid-1.0.12-release.apk
_ ____ _ ___ _
/ \ | _ \| |/ / | ___ __ _| | _____
/ _ \ | |_) | ' /| | / _ \/ _` | |/ / __|
/ ___ \| __/| . \| |__| __/ (_| | <\__ \
/_/ \_\_| |_|\_\_____\___|\__,_|_|\_\___/
v2.6.3
--
Scanning APK file for URIs, endpoints & secrets
(c) 2020-2024, dwisiswant0
Can't find jadx binary.
Do you want to download jadx? (Y/n) Y
** Downloading jadx...
** Decompiling APK...
INFO - loading ...
INFO - processing ...
ERROR - finished with errors, count: 2
** Scanning against 'b3nac.injuredandroid'
[Firebase]
- injuredandroid.firebaseio.com
[Google_API_Key]
- AIzaSyCUImEIOSvqAswLqFak75xhskkB6illd7A
[Google_Cloud_Platform_OAuth]
- 430943006316-85ibmlobpn5p6c14b2keslrh5r6kgsn4.apps.googleusercontent.com
[JSON_Web_Token]
- 1.1.0-alpha01
- 1.6.0-rc01
- version=16.0.0
- version=17.0.0
- version=17.1.0
- version=19.0.0
- version=19.2.0
Manifest Files
The Android Manifest is an XML file which contains metadata about the application. This includes the package name, activity names, main activity, supported Android version and permissions.
Reviewing the InjuredAndroid manifest file, we can see it has the ability to access the Internet and write to storage.
cat AndroidManifest.xml | grep permission
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.READ_PHONE_STATE"/>
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
In addition, the application contains exported activities that other applications on the phone may be able to interact with. An activity is a user interface window the application uses.
cat AndroidManifest.xml | grep -i "activity android:exported"
<activity android:exported="true" android:label="@string/title_activity_flag_eighteen" android:name="b3nac.injuredandroid.FlagEighteenActivity" android:theme="@style/AppTheme.NoActionBar"/>
<activity android:exported="true" android:label="@string/title_activity_exported_protected_intent" android:name="b3nac.injuredandroid.ExportedProtectedIntent" android:theme="@style/AppTheme.NoActionBar"/>
<activity android:exported="true" android:name="b3nac.injuredandroid.QXV0aA"/>
<activity android:exported="true" android:name="b3nac.injuredandroid.b25lActivity"/>
<activity android:exported="true" android:label="@string/title_activity_test_broadcast_reciever" android:name="b3nac.injuredandroid.TestBroadcastReceiver" android:theme="@style/AppTheme.NoActionBar"/>
<activity android:exported="false" android:name="com.google.android.gms.common.api.GoogleApiActivity" android:theme="@android:style/Theme.Translucent.NoTitleBar"/>
We can call exported activities using adb shell, which can potential allow us to access parts of the application the developer did not intend.
adb shell am start -n b3nac.injuredandroid/.b25lActivity
Starting: Intent { cmp=b3nac.injuredandroid/.b25lActivity }
SQLite Databases
Many Android applications use SQLite for database storage. These might contain interesting information. You can use ADB to extract SQLite databases.
user@host:~$ adb root
restarting adbd as root
emulator64_x86_64_arm64:/ # pm list packages | grep injuredandroid
package:b3nac.injuredandroid
emulator64_x86_64_arm64:/ # ls -R /data/data/b3nac.injuredandroid/databases/
/data/data/b3nac.injuredandroid/databases:
Thisisatest.db Thisisatest.db-journal
adb pull /data/data/b3nac.injuredandroid/databases/Thisisatest.db
/data/data/b3nac.injuredandroid/databases/Thisisatest.db: 1 file pulled, 0 skipped. 0.4 MB/s (16384 bytes in 0.042s)
Information can then be extracted from the database either using a command line or GUI SQLite browser. The clients can be installed using;
sudo apt install sqlitebrowser sqlite3
We can then review the database contents.
sqlite3 Thisisatest.db
SQLite version 3.45.1 2024-01-30 16:01:20
Enter ".help" for usage hints.
sqlite> .tables
Thisisatest android_metadata
sqlite> select * from Thisisatest;
1|The flag hash!|2ab96390c7dbe3439de74d0c9b0b1767
2|The flag is also a password!|9EEADi^^:?;FC652?5C@:5]7:C632D6:@]4@>^DB=:E6];D@?
Insecure Local Storage
Android offers the SharedPreferences API to allow developers to easily store key-value pair information. Information recorded using the API is stored in plaintext XML files. Using adb shell we can view the contents of these files.
emulator64_x86_64_arm64:/ # ls -R /data/data/b3nac.injuredandroid/
/data/data/b3nac.injuredandroid/:
cache databases shared_prefs
emulator64_x86_64_arm64:/ # cat shared_prefs/b3nac.injuredandroid_preferences.xml
<?xml version='1.0' encoding='utf-8' standalone='yes' ?>
<map>
<string name="username">bordergate</string>
<string name="password">bordergate</string>
</map>
Firebase Databases
Firebase is a cloud hosted NoSQL Database that is frequently used in Android applications. Searching strings.xml for “firebase” should show firebase URL’s in the following format:
<nameofdatabase>.firebaseio.com
Firebase access control rules may be misconfigured allowing for unrestricted read access. To test for this, attempt to access the .json file.
curl -x GET https://nameofdatabase.firbaseio.com/.json
Overly Verbose Log Messages
Android applications can use the Log API for debugging logging. Applications can potentially write sensitive information into these log messages. The adb logcat command can be used to view these messages. This output can be piped to grep to find potentially sensitive information.
adb logcat | grep -i "password|key|token|secret"
Certificate Pinning
Many Android applications implement certificate pinning to prevent traffic interception. You can bypass certificate pinning using objection. I’ve previously discussed how to do this in the following article.
With certificate pinning bypassed, you can examine calls the application makes to web services using an intercepting proxy such as BurpSuite.
MOBSF
MobSF is an automated dynamic analysis system for mobile applications, which is useful for quickly security issues. Information on setting this up can be found in this article.
In Conclusion
Many android applications are simply interfaces to online services, with some local data storage. As such, the majority of threats relate to web application vulnerabilities, and insecure usage of local data storage.